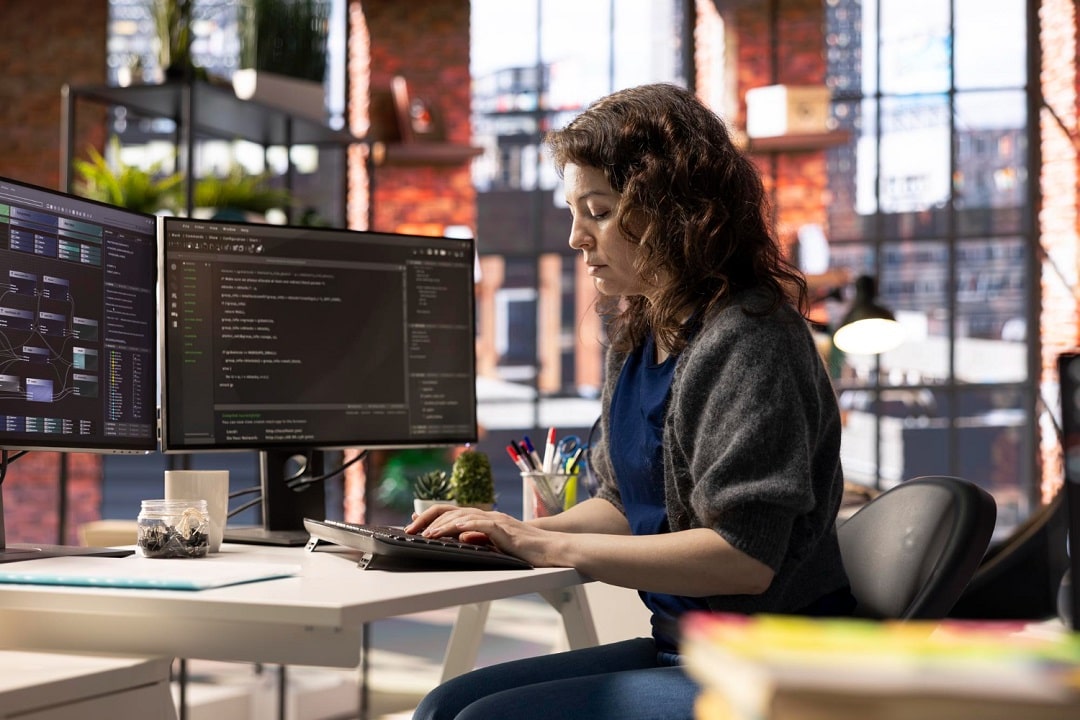
Polymorphism is one of the fundamental concepts in OOP that provides flexibility and reusability to the design of object-oriented software. For a beginner entering the realm of programming, it may be overwhelming to learn what is polymorphism. However, once an understanding is formed, it becomes a weapon for creating scalable and maintainable applications.
This article will try to describe polymorphism in OOP in simple words. It will teach you what it is, what the types are, and how polymorphism is used in languages such as Java, Python, and C++.
Introduction to Object-Oriented Programming
Object-Oriented Programming (OOP) refers to a programming paradigm that structures the code into several objects that stand for many real-world or existing entities. Such objects will encapsulate data and behavior, thereby making the code relatively easier to manage, test, and reuse.
The four pillars of OOP are:
- Encapsulation
- Inheritance
- Abstraction
- Polymorphism
Let’s now dive deeper into what is polymorphism and why it’s vital in OOP.
What is Polymorphism?
The term polymorphism comes from the Greek word, where “poly” means many and “morph” refers to form. In a programming context, polymorphism in OOP refers to different classes being treated as instances of the same class through a common interface.
Polymorphism signifies the ability of a single method, function, or operator to behave differently concerning different objects. This is the basis for the dynamism and adaptability of code.
Example:
class Animal:
def speak(self):
return “Animal speaks”
class Dog(Animal):
def speak(self):
return “Dog barks”
class Cat(Animal):
def speak(self):
return “Cat meows”
def make_sound(animal):
print(animal.speak())
make_sound(Dog()) # Outputs: Dog barks
make_sound(Cat()) # Outputs: Cat meows
Here, make_sound can take any object of type Animal or its subclass. This is polymorphism in OOP in action.
Why Polymorphism in OOP is Important
Understanding what is polymorphism helps developers create code that is:
- Extensible – Easily add new classes or methods.
- Maintainable – Reduce code duplication.
- Scalable – Accommodate future changes.
- Clean and DRY (Don’t Repeat Yourself) – One interface, multiple implementations.
Polymorphism enhances the overall design and readability of software applications.
Types of Polymorphism
There are two major types of polymorphism in object-oriented programming:
- Compile-Time Polymorphism (Static Polymorphism)
- Runtime Polymorphism (Dynamic Polymorphism)
Let’s explore each type in detail.
1. Compile-Time Polymorphism (Static Polymorphism)
Compile-time polymorphism occurs when the method to be invoked is determined at compile time. It is achieved using:
- Method Overloading
- Operator Overloading
Method Overloading:
This means creating multiple methods with the same name but different parameters within the same class.
Example in Java:
class Calculator {
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
}
Both add methods perform addition but accept different data types. This is compile-time polymorphism.
Operator Overloading (in languages like C++):
class Complex {
public:
int real, imag;
Complex operator + (Complex const &obj) {
Complex res;
res.real = real + obj.real;
res.imag = imag + obj.imag;
return res;
}
};
Here, the + operator behaves differently based on the operands – a form of polymorphism in OOP.
2. Runtime Polymorphism (Dynamic Polymorphism)
In runtime polymorphism, the method to be invoked is determined during program execution. It is achieved via method overriding using inheritance.
Method Overriding:
Occurs when a subclass provides a specific implementation of a method already defined in its parent class.
Example in Python:
class Vehicle:
def move(self):
print(“Vehicle is moving”)
class Car(Vehicle):
def move(self):
print(“Car is driving”)
v = Vehicle()
c = Car()
v.move() # Outputs: Vehicle is moving
c.move() # Outputs: Car is driving
This is runtime polymorphism, where the method call is resolved at runtime depending on the object’s actual class.
Polymorphism in Different Programming Languages
Polymorphism in OOP is implemented differently across languages:
- Java: Method overloading is an example of compile-time polymorphism, and overriding is an example of runtime polymorphism.
- Python: Duck typing provides implicit polymorphism without strict type checks.
- C++: strong support for both operator overloading and virtual functions.
- C#: Just like Java, it also supports method overloading and overriding using keywords such as virtual and override.
Understanding how each language implements polymorphism in OOP can help write more idiomatic code.
Real-World Examples of Polymorphism in OOP
Example 1: Payment System
class Payment:
def process(self):
raise NotImplementedError
class CreditCard(Payment):
def process(self):
print(“Processing credit card payment”)
class PayPal(Payment):
def process(self):
print(“Processing PayPal payment”)
def checkout(payment_method):
payment_method.process()
checkout(CreditCard()) # Outputs: Processing credit card payment
checkout(PayPal()) # Outputs: Processing PayPal payment
The checkout function can process any type of payment – credit card, PayPal, or even others added later. This is the power of polymorphism in OOP.
Example 2: GUI Framework
A button, slider, and checkbox might all have a method called draw(). Even though they are different elements, a single interface can be used to render them all dynamically.
Advantages of Using Polymorphism
- Reusability of Codes: Reuse an existing set of codes with little or no modification.
- Complexity Reduction: The total Object Interaction is simplified.
- Better Maintainability: Easy to upgrade or incorporate new functionalities.
- Flexible Architecture: A system’s adaptation to change is improved.
Common Misconceptions
- Polymorphism means method overloading only – Wrong. It also includes method overriding and operator overloading.
- It slows down performance – Not really. Most compilers, as well as interpreters, are optimized for polymorphic behavior.
- Polymorphism is not mandatory – Technically, yes. But it is a necessity of good object-oriented design.
Conclusion
Polymorphism in OOP is not just a buzzword; it is one of the very potent programming principles based on which flexible and extensible clean code is possible. Whether this would be a bank-related application or a mobile application, or a game engine built using object-oriented principles, incorporating polymorphic behavior allows developing the required adaptable software solution for easier maintenance and scaling purposes.
Studying polymorphism, its different types, and their practical applications will aid developers in writing clean and efficient code. Both compile-time and run-time polymorphism have their usages and provide scope to a perfect aspiring software engineer in their journey.